Greetings and welcome to the first episode of THE ROAD TO AI, where I document my humble efforts in understanding the intricate simplicity of Artificial Intelligence.
I guess my journey begins back in the university in a class called “Numeric Algebra”. In that class we had learned to compute, solve and approximate continuous functions using discrete methods.
One of the cooler things we’d learned was the function interpolation using backpropagation.
As I saw that one could actually predict data points, I immediately thought to my self “I wonder if that’s how AI’s operate?”.
Two years later I found myself in need of building a particle physics engine. Conceptually, its simple – All I had to do was to create a “world” which contained particles in the shape of small circles and simulate the interactions between them, and between them and outside forces. Needless to say, doing that elegantly was not that trivial.
Searching for answers in the deep realm of YouTube – I found out about this amazing guy named Daniel Shiffman who hosted a channel called “The Coding Train“. Daniel is a very nice, humble and unbelievably awesome teacher. He teaches several programming languages and environments such as the following:
- Processing – a Java based framework that simplifies the creating of visual animation and other sorts of art
- P5js – The same as processing but for web based applications. This language is JavaScript based.
- TensorFlow.js – A huge library developed by Google to quickly deploy ML algorithms usually within a cloud computing service.
- ML5js – A friendly machine learning library for web apps. Based on Tensorflow.js
- GIT – The ins and outs of GitHub
He also teaches Cabana, Html, and other cool tutorials like working with data, API’s and such.
I went through the whole series of “The Nature Of The Code” in which he follows a whole semester’s curriculum programming a physics engine from particle interactions, to planetary motion, ending with Genetic Algorithms, Neural Networks and Neuroevolution.
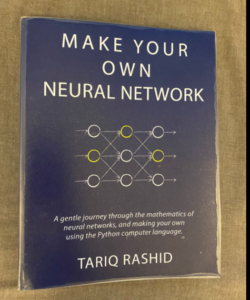
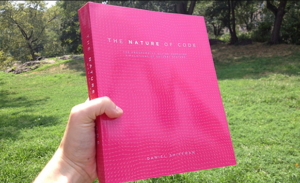
I was SO fascinated by this course, I had even bought the book Daniel had written on the subject. It’s pay as you will, free for download and everything, but I did end up paying to support his work. In the books and the Youtube series he also references a book named – “Make Your Own Neural Network” by Tariq Rashid; Where Tariq teaches the math around the concept of Neural Networks, and then walks the reader through writing their own one in Python. Of course, I had bought that one too 😀
So after giving my sort-of online mentor the proper credit and introduction, lets move on to one of my first projects:
FLOCKING(Boids) SIMULATION
The Boids algorithm, developed by Craig W. Reynolds, is used to simulate the flocking behavior of birds. The beauty of it is in its simplicity.
The algorithm consists of a population of agents where each individual agent able to perceive its surroundings, is forced to abide theses three rules:
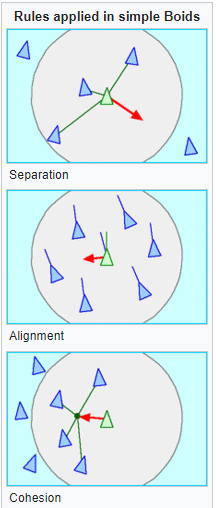
- Separation –
Each agent should avoid interfering with its local neighbors. - Alignment –
Each agent should align to average velocity of its local neighbors. - Cohesion –
Each agent should strive for the average position of its local neighbors.
In Atom IDE , I created two new classes –
class Agent{}
class AgentSystem{}
The AgentSystem class was implemented as a separate entity to handle all of the agents.
The Agent class had the three methods – align(agentsList){}
cohese(agentsList){}
separate(agentsList){}
The alignment was the easy part –
Then the Cohesion, which is almost the same but rather then averaging over the velocities, we need to average over the positions, attain the vector pointing from the agent to the desired position, then continue as in the alignment –
Lastly – We need to implement the separation algorithm. Here we calculate the average vector pointing from the agent to its neighbors, multiplying by -1 and we get the direction in which we need to go. We also apply a scaling factor to increase the “need” to run faster from closer agents.
And VOILA! we have the flocking simulation right here for your convenience:
Feel free to adjust the force factor of each of the three rules, the perception radius of each agent, or the separation distance, and see how the complex population interacts –
A few optimizations I should do –
- I could condense the three methods together, so that I only needed to iterate over all the other agents once for each agent instead of three times. I intestinally did that for my own educational purposes.
- I could divide the whole space into squares of sum size so that for each agent I would only have to iterate over the neighbors in its square.
- I could also narrow each of the agents field of view, so that it could only “see” a certain degrees in front of it, instead of the hole radius surrounding it.
- Something cool to try would be to set a perception radius for each one of the different rules
Anyway, I hope you enjoyed this post just as I enjoyed writing this program.
Stay tuned for the next episode of THE ROAD TO AI.
Until then, Refactor life as you please